Electronics projects for kids: How to create an Arduino timer with a seven-segment display
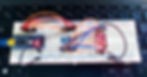
Hi there!!!
Welcome to Makersgeneration, your go-to destination for cool STEM and electronics projects for kids.
If you are a regular on this platform, then you are not new to the Arduino development platform. For newcomers, Arduino helps us bring all our electronic gadget ideas to life. It is an excellent platform that comes in handy when exploring and learning about electronics and programming for kids. Arduino offers a simple interface and a wide range of sensors with endless possibilities for creative projects.
For today's project, we will learn how to make a simple Arduino timer using Seven Segment Display. By the end of this project, you should now understand how those numbers on your calculator or how the time on your digital watch is being displayed.
Seven Segment Display
A seven-segment display is a form of electronic display device used for displaying numbers and sometimes letters. It consists of seven individually illuminated segments arranged in a rectangular pattern, typically in the form of an "8" or a square. Each segment is labeled from "a" to "g", and by selectively turning on or off these segments, various numbers (0-9) and some letters (A-F) can be displayed.
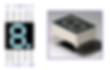
.
To display a specific digit, you would activate or illuminate the required segments by providing the appropriate electrical signals. For example, to display the number "1", you would typically activate segments "b" and "c" while keeping the other segments turned off.

Seven-segment displays are commonly used in digital clocks, calculators, and other electronic devices where numerical or alphanumeric information needs to be visually presented. They can be found in various sizes, from small individual digits to larger multi-digit displays.
Modern versions of seven-segment displays may incorporate additional features such as decimal points, colons (for time displays), or extra segments for displaying letters or special symbols.
Required components
Arduino Nano or Uno
Common-cathode Seven Segment Display
50 - 330 ohms resistors (7)
Male-Male jumper wires (16)
Breadboard
Compatible USB cable
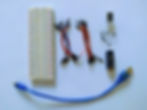
.
Before we get into building the circuit, let's take a look at the two major types of seven-segment displays.
Types of Seven-Segment Displays
Seven-segment displays are mainly categorized into two types, Common Cathode (CC) and Common Anode (CA).
Common Anode (CA) Seven-Segment Display: In this type, all the anodes of the individual segments are connected together and connected to the positive power supply. The cathodes of each segment are separate and connected to the ground or logic level to illuminate them.
Common Cathode (CC) Seven-Segment Display: In this type, all the cathodes of the individual segments are connected together and grounded. The anodes of each segment are separate and connected to the positive power supply to illuminate them.
Building the circuit
We will be using a Common cathode seven-segment display in this project, which means the two com pins will be connected to the ground of the Arduino, while the other pins will be connected to designated Arduino digital pins via resistors, as in the circuit below:
Since Arduino digital pins output 5v which can damage the LED on the seven-segment display, resistors are used to step down the current - You can use resistors within the range of 50 - 330 ohms.
...We will ignore the (dot) pin - as we will not need it in this project.



Arduino timer code
void setup(){
// initialize serial communication
Serial.begin(9600);
// Arduino pins for connecting SSD
pinMode(2, OUTPUT); //pin a
pinMode(3, OUTPUT); //pin b
pinMode(4, OUTPUT); //pin c
pinMode(5, OUTPUT); //pin d
pinMode(6, OUTPUT); //pin e
pinMode(7, OUTPUT); //pin f
pinMode(8, OUTPUT); //pin g
}
void loop(){
// digit 0
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, HIGH):
digitalWrite(6, HIGH):
digitalWrite(7, HIGH):
digitalWrite(8, LOW):
delay(1000);
// digit 1
digitalWrite(2, LOW):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, LOW):
digitalWrite(6, LOW):
digitalWrite(7, LOW):
digitalWrite(8, LOW):
delay(1000);
// digit 2
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, LOW):
digitalWrite(5, HIGH):
digitalWrite(6, HIGH):
digitalWrite(7, LOW):
digitalWrite(8, HIGH):
delay(1000);
// digit 3
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, HIGH):
digitalWrite(6, LOW):
digitalWrite(7, LOW):
digitalWrite(8, HIGH):
delay(1000);
// digit 4
digitalWrite(2, LOW):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, LOW):
digitalWrite(6, LOW):
digitalWrite(7, HIGH):
digitalWrite(8, HIGH):
delay(1000);
// digit 5
digitalWrite(2, HIGH):
digitalWrite(3, LOW):
digitalWrite(4, HIGH):
digitalWrite(5, HIGH):
digitalWrite(6, LOW):
digitalWrite(7, HIGH):
digitalWrite(8, HIGH):
delay(1000);
// digit 6
digitalWrite(2, HIGH):
digitalWrite(3, LOW):
digitalWrite(4, HIGH):
digitalWrite(5, HIGH):
digitalWrite(6, HIGH):
digitalWrite(7, HIGH):
digitalWrite(8, HIGH):
delay(1000);
// digit 7
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, LOW):
digitalWrite(6, LOW):
digitalWrite(7, LOW):
digitalWrite(8, LOW):
delay(1000);
// digit 8
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, HIGH):
digitalWrite(6, HIGH):
digitalWrite(7, HIGH):
digitalWrite(8, HIGH):
delay(1000);
// digit 9
digitalWrite(2, HIGH):
digitalWrite(3, HIGH):
digitalWrite(4, HIGH):
digitalWrite(5, LOW):
digitalWrite(6, LOW):
digitalWrite(7, HIGH):
digitalWrite(8, HIGH):
delay(1000);
}
How code works:
The code above is pretty to understand.
Firstly, inside the void setup() - we initialize the serial communication between the board and the computer. Next, we declare the Arduino pins to which the Seven segment display will be connected and set the signal to OUTPUT (i.e send out 5V to light the LEDs)
Secondly, in the void loop() - using the digitalWrite(pin number, signal) command, we tell the Arduino which pins to output 5V at a time to light up the desired LED to form the number we want on the seven-segment display for about 1 second (delay(1000)).
In the loop, each number will display for 1 second then move to the next number until it gets to the end of the loop (0,1,2...9), then start again from the beginning.
To run the code:
Open the Arduino IDE, copy the code above, and paste it into the IDE's open window.
Navigate to Tools >> Select Board type (Arduino Uno or Nano) >> Select the correct Port assigned to your board.

Demo video
Online after-school and summer camps in Silver Spring Maryland
You and your children are looking for nice activities to have fun and learn new things and skills. Come join us starting in May and this summer for more online classes such as:
Python coding for kids and teens
Coding for elementary school students
Make video games
Electronics
Digital modeling (Create cars, rockets, rings, etc) for 3D printing and much more with the following link: Online after-schools
And the STEM summer camp for your kids is organized in Silver Spring Maryland nearby Washington DC to have fun and learn more about robotics, coding, droning, and more. Check the link right here:
Other cute things to make and hand-crafts for kids
If you are looking for more cool Arduino and STEM project ideas to do with your kids, take a look at these other activities:
Newsletter, follow, subscribe, and like the social media
If you like online STEM activities, consider subscribing to the newsletter and social media for updates, and don't miss any STEM events. Don't forget to subscribe to the newsletter at the bottom of our website ''www.makersgeneration.net'' for more events, tutorials, and freebies.
Subscribe to the Facebook group if you have yet registered. Content and tutorials are shared daily: Create and build STEM projects for kids
We can be reached at: contact@makersgeneration.net if any questions.
See you on soon.